Evolution of Scaling & High Availability in Web Development
One of the most significant challenges in web development has been achieving scale and high availability as applications grow in popularity. This article traces the evolution of scaling strategies from the early days of standalone servers to modern distributed systems architecture, exploring how we've arrived at today's complex landscape of scalability solutions.
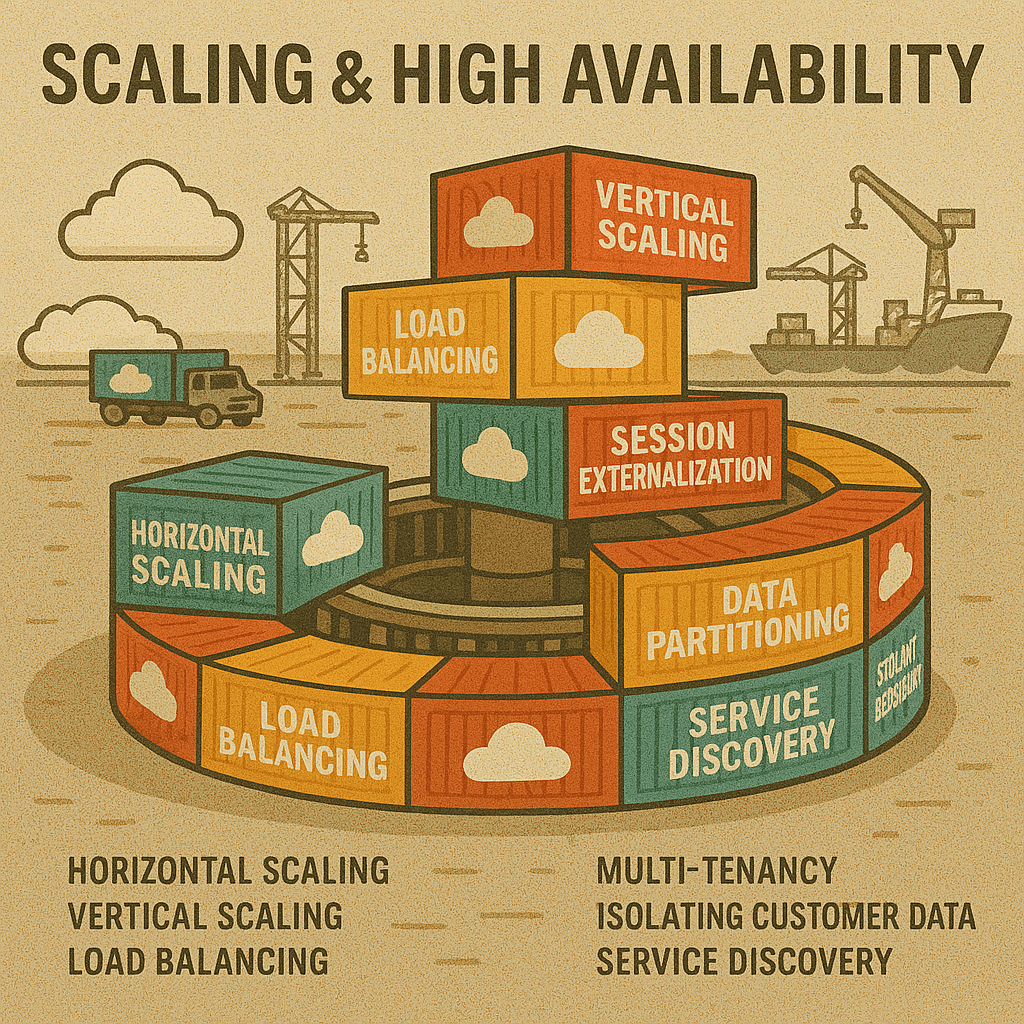
The evolution of web scaling architectures from monolithic servers to distributed cloud systems
The Early Days: Vertical Scaling (1990s-Early 2000s)
In the early web era, scaling primarily meant upgrading your single server - "scaling up" rather than "scaling out." When traffic increased, administrators would add more CPU, RAM, or disk space to the existing machine.
Early Scaling Approach: The "Bigger Iron" Solution
# Typical server upgrade order in the late 1990s
1. Start with entry-level server
2. Add more RAM
3. Add faster/more CPUs
4. Upgrade to better I/O subsystem
5. Eventually replace with entirely new, more powerful server
# Common server specs evolution
1995: Single Pentium, 32MB RAM, single IDE disk
1998: Dual Pentium II, 256MB RAM, SCSI disk array
2001: Quad Xeon, 4GB RAM, hardware RAID, SAN storage
This approach had significant limitations. There was a physical ceiling to how much a single machine could scale, and hardware upgrades meant system downtime. The entire application ran on a single server, creating a single point of failure.
The Missed Opportunity: Integrated Application Servers
Looking back, we can identify a path not taken that might have simplified today's complex scaling landscape. Early application servers like WebLogic, WebSphere, and JBoss offered built-in clustering capabilities with relatively simple configuration.
WebLogic Clustering Configuration (circa 2003)
Instead of continuing to evolve these integrated platforms, the industry moved toward decomposition and specialization. This created more flexibility but also significantly more complexity, as developers now had to assemble and configure multiple specialized components rather than working with a pre-integrated platform.
The Transition: Load Balancing & Early Horizontal Scaling (Early-Mid 2000s)
As web applications grew more complex and traffic increased, developers began embracing horizontal scaling - adding more servers rather than making individual servers more powerful. This approach required the introduction of load balancers to distribute traffic.
Apache Load Balancer Configuration (Early 2000s)
# Apache mod_proxy configuration for basic load balancing
BalancerMember http://app1.example.com:8080
BalancerMember http://app2.example.com:8080
ProxySet lbmethod=byrequests
ProxyPass /app balancer://mycluster/app
ProxyPassReverse /app balancer://mycluster/app
# Session affinity was achieved using "sticky sessions"
BalancerMember http://app1.example.com:8080 route=app1
BalancerMember http://app2.example.com:8080 route=app2
ProxySet stickysession=JSESSIONID
Early horizontal scaling introduced new challenges. Sticky sessions helped maintain user context across requests, but they undermined the benefits of load balancing and created issues when servers went down. State management became a critical concern.
Stateless Applications & Shared Database (Mid-2000s)
To address the limitations of sticky sessions, developers started building more stateless applications. User sessions and application state moved from in-memory storage to shared databases, allowing any application server to handle any request.
PHP Session Database Storage (Mid-2000s)
# php.ini configuration for database session storage
session.save_handler = user
session.save_path = "mysql:host=db.example.com;dbname=sessions"
# Custom session handler class
class DBSessionHandler {
private $db;
public function open($path, $name) {
$this->db = new PDO('mysql:host=db.example.com;dbname=sessions', 'user', 'password');
return true;
}
public function read($id) {
$stmt = $this->db->prepare("SELECT data FROM sessions WHERE id = ? AND expiry > ?");
$stmt->execute(array($id, time()));
$data = $stmt->fetchColumn();
return $data === false ? '' : $data;
}
public function write($id, $data) {
$stmt = $this->db->prepare("REPLACE INTO sessions (id, data, expiry) VALUES (?, ?, ?)");
return $stmt->execute(array($id, $data, time() + 3600));
}
// Other methods: close, destroy, gc...
}
# Register the handler
$handler = new DBSessionHandler();
session_set_save_handler(
array($handler, 'open'), array($handler, 'close'),
array($handler, 'read'), array($handler, 'write'),
array($handler, 'destroy'), array($handler, 'gc')
);
While this approach improved scalability, it introduced a new bottleneck: the database. As applications scaled further, even powerful database servers struggled to handle session loads, creating a new single point of failure.
Distributed Caching & Memory Grids (Late 2000s-Early 2010s)
To address database bottlenecks, developers began adopting distributed caching systems like Memcached and, later, Redis. These systems provided fast, in-memory access to session data and other frequently accessed information without the overhead of database transactions.
Rails Cache Configuration with Memcached (Late 2000s)
# config/environments/production.rb
Rails.application.configure do
# Configure Memcached as the cache store
config.cache_store = :mem_cache_store,
"memcache1.example.com:11211",
"memcache2.example.com:11211",
{
namespace: "myapp",
expires_in: 1.day,
compress: true,
failover: true
}
# Store sessions in Memcached
config.session_store :mem_cache_store,
servers: ["memcache1.example.com:11211", "memcache2.example.com:11211"],
key: "_myapp_session",
expire_after: 1.day
end
# Using the cache in application code
class ProductsController < ApplicationController
def index
@top_products = Rails.cache.fetch("top_products", expires_in: 1.hour) do
Product.top_sellers.to_a
end
end
end
Distributed caching improved performance dramatically but added a new layer of complexity to the application stack. Developers now had to manage cache invalidation, handle cache misses, and ensure data consistency between the cache and the authoritative data store.
Cloud Scaling & Elastic Infrastructure (2010s)
The rise of cloud computing brought elastic infrastructure - the ability to automatically add or remove servers based on demand. This capability fundamentally changed how applications scaled, enabling dynamic resource allocation and significant cost optimization.
AWS Auto Scaling Configuration (2010s)
# AWS CloudFormation template excerpt for Auto Scaling Group
Resources:
WebServerGroup:
Type: AWS::AutoScaling::AutoScalingGroup
Properties:
AvailabilityZones:
- us-east-1a
- us-east-1b
- us-east-1c
LaunchConfigurationName: !Ref WebServerLaunchConfig
MinSize: 2
MaxSize: 10
DesiredCapacity: 2
HealthCheckType: ELB
HealthCheckGracePeriod: 300
TargetGroupARNs:
- !Ref WebServerTargetGroup
Tags:
- Key: Name
Value: web-server
PropagateAtLaunch: true
# Auto Scaling policy based on CPU utilization
WebServerScaleUpPolicy:
Type: AWS::AutoScaling::ScalingPolicy
Properties:
AdjustmentType: ChangeInCapacity
AutoScalingGroupName: !Ref WebServerGroup
Cooldown: 300
ScalingAdjustment: 1
CPUAlarmHigh:
Type: AWS::CloudWatch::Alarm
Properties:
AlarmDescription: Scale up when CPU > 70% for 5 minutes
MetricName: CPUUtilization
Namespace: AWS/EC2
Statistic: Average
Period: 300
EvaluationPeriods: 1
Threshold: 70
AlarmActions:
- !Ref WebServerScaleUpPolicy
Dimensions:
- Name: AutoScalingGroupName
Value: !Ref WebServerGroup
ComparisonOperator: GreaterThanThreshold
Cloud scaling facilitated unprecedented flexibility but introduced new complexities around infrastructure management. To address this, the industry developed Infrastructure as Code (IaC) practices, treating server configurations as software to be versioned, tested, and deployed automatically.
Containerization & Orchestration (Mid 2010s-Present)
The container revolution, led by Docker and orchestrated by systems like Kubernetes, brought new approaches to application packaging and deployment. Containers provided consistent environments across development and production while improving resource utilization.
Kubernetes Deployment Configuration
# Kubernetes deployment manifest for a scalable web application
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-application
labels:
app: web
spec:
replicas: 3
selector:
matchLabels:
app: web
strategy:
type: RollingUpdate
rollingUpdate:
maxUnavailable: 1
maxSurge: 1
template:
metadata:
labels:
app: web
spec:
containers:
- name: web-app
image: example/web-app:v1.2.3
ports:
- containerPort: 8080
resources:
requests:
memory: "256Mi"
cpu: "100m"
limits:
memory: "512Mi"
cpu: "500m"
readinessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 10
periodSeconds: 5
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 15
periodSeconds: 15
# Horizontal Pod Autoscaler for automatic scaling
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: web-application-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: web-application
minReplicas: 3
maxReplicas: 20
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 70
Container orchestration platforms automated many aspects of application deployment and scaling, but they also added significant complexity. Many organizations found they needed dedicated platform teams to manage their Kubernetes clusters effectively.
Microservices & Distributed Systems (2015-Present)
The microservices architecture pattern decomposed monolithic applications into smaller, independently deployable services. This approach aligned well with container orchestration, allowing teams to scale individual components of their application independently.
Microservices Communication with Service Mesh
# Istio service mesh configuration
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: user-service
spec:
hosts:
- user-service
http:
- route:
- destination:
host: user-service
subset: v1
weight: 90
- destination:
host: user-service
subset: v2
weight: 10
retries:
attempts: 3
perTryTimeout: 2s
timeout: 5s
# Circuit breaker configuration
apiVersion: networking.istio.io/v1alpha3
kind: DestinationRule
metadata:
name: user-service
spec:
host: user-service
trafficPolicy:
connectionPool:
http:
maxRequestsPerConnection: 10
http1MaxPendingRequests: 1024
tcp:
maxConnections: 100
outlierDetection:
consecutiveErrors: 3
interval: 5s
baseEjectionTime: 30s
subsets:
- name: v1
labels:
version: v1
- name: v2
labels:
version: v2
Microservices offered improved team autonomy and scalability but introduced complex problems in distributed systems: service discovery, load balancing, circuit breaking, retries, and more. Service meshes like Istio emerged to handle these challenges, adding yet another layer of infrastructure complexity.
The Path Not Taken: Integrated App Servers as Cloud Platforms
When we examine the evolution of web scaling, it's interesting to consider what might have been. The application servers of the early 2000s (WebLogic, WebSphere, etc.) already had many of the capabilities we now implement through complex orchestration:
- Built-in clustering and load balancing
- Distributed session replication
- Health monitoring and automatic failover
- Connection pooling and resource management
Had these platforms evolved to embrace cloud-native patterns and container technology, we might have seen a world where application servers themselves became the cloud platform - offering built-in replication, auto-scaling, and high availability with simple configuration rather than complex orchestration.
What Could Have Been: Hypothetical Cloud-Native App Server
# Hypothetical configuration for an evolved app server platform
app:
name: my-web-application
version: 1.2.3
scaling:
min_instances: 3
max_instances: 20
metrics:
- type: cpu
target_utilization: 70
- type: requests_per_second
target_value: 1000
scaling_policy:
cool_down: 300s
deployment:
strategy: rolling
max_unavailable: 1
max_surge: 1
health_check:
path: /health
initial_delay: 10s
period: 5s
session:
storage: distributed
replication: synchronous
timeout: 30m
resilience:
circuit_breaker:
enabled: true
failure_threshold: 5
reset_timeout: 30s
retry:
attempts: 3
backoff: exponential
resources:
memory:
min: 256Mi
max: 512Mi
cpu:
min: 0.1
max: 0.5
Instead, the industry moved toward specialized components written in diverse languages, often requiring more CPU, memory, and storage than integrated platforms. This specialization brought flexibility but at the cost of increased complexity and resource utilization.
Serverless & Function-as-a-Service (Late 2010s-Present)
The serverless paradigm emerged as a reaction to the growing complexity of container orchestration. It abstracted away infrastructure management entirely, allowing developers to focus solely on their application's business logic.
AWS Lambda Function with API Gateway Integration
# AWS SAM template for a serverless API
AWSTemplateFormatVersion: '2010-09-09'
Transform: 'AWS::Serverless-2016-10-31'
Resources:
UserFunction:
Type: 'AWS::Serverless::Function'
Properties:
CodeUri: ./user-service/
Handler: index.handler
Runtime: nodejs14.x
MemorySize: 128
Timeout: 3
AutoPublishAlias: live
DeploymentPreference:
Type: Canary10Percent5Minutes
Events:
GetUsers:
Type: Api
Properties:
Path: /users
Method: get
GetUser:
Type: Api
Properties:
Path: /users/{userId}
Method: get
CreateUser:
Type: Api
Properties:
Path: /users
Method: post
Environment:
Variables:
TABLE_NAME: !Ref UsersTable
Policies:
- DynamoDBCrudPolicy:
TableName: !Ref UsersTable
UsersTable:
Type: 'AWS::DynamoDB::Table'
Properties:
AttributeDefinitions:
- AttributeName: userId
AttributeType: S
KeySchema:
- AttributeName: userId
KeyType: HASH
BillingMode: PAY_PER_REQUEST
Serverless computing offered unprecedented scaling capabilities - automatically handling requests from zero to thousands per second without any configuration. However, it introduced challenges around cold starts, execution limits, and vendor lock-in.
Edge Computing & Global Distribution (Present-Future)
The latest evolution in scaling is moving computation closer to users through edge computing. Content delivery networks (CDNs) have evolved into edge computation platforms, allowing code execution at global edge locations.
Cloudflare Workers Edge Function
// Cloudflare Worker serving content from the edge
addEventListener('fetch', event => {
event.respondWith(handleRequest(event.request))
})
async function handleRequest(request) {
// Get user's geolocation from request headers
const country = request.headers.get('CF-IPCountry')
// Get data from globally distributed KV store
const userData = await USERS_KV.get(request.url.split('/').pop(), 'json')
if (!userData) {
return new Response('User not found', { status: 404 })
}
// Customize content based on user location
if (country === 'DE') {
userData.greeting = 'Hallo'
} else if (country === 'FR') {
userData.greeting = 'Bonjour'
} else {
userData.greeting = 'Hello'
}
// Return localized response from the edge
return new Response(JSON.stringify(userData), {
headers: { 'Content-Type': 'application/json' }
})
}
Edge computing brings content and computation closer to users, reducing latency and improving resilience through global distribution. However, it introduces new challenges around data consistency, cold starts at the edge, and complex debugging across a global network.
The Current State: Complexity and Specialization
Today's web scaling landscape is characterized by unprecedented flexibility but also extraordinary complexity. A typical modern web application stack might include:
- Edge CDN for static content and edge functions
- Global load balancers
- Regional Kubernetes clusters
- Service mesh for inter-service communication
- Distributed databases (often multiple types for different data patterns)
- Distributed caching systems
- Message queues for asynchronous processing
- Monitoring and observability platforms
- CI/CD pipelines for continuous deployment
Each component solves specific problems, but the integration of these components creates a system whose complexity far exceeds that of earlier generations of web platforms.
Emerging Trends: Simplification and Integration
In response to growing complexity, the industry is showing signs of seeking simplification and re-integration. We're seeing:
- Platform Engineering teams creating internal developer platforms to abstract complexity
- Managed Kubernetes services that hide infrastructure details
- Serverless container platforms that combine container flexibility with serverless simplicity
- Application frameworks with built-in distribution capabilities
- Database systems incorporating cache, search, and event streaming capabilities
These trends suggest we may see a pendulum swing back toward integrated platforms, albeit ones built on the lessons learned from cloud-native architecture.
Modern Internal Developer Platform
# Platform configuration hiding infrastructure complexity
application:
name: customer-portal
team: customer-experience
components:
- name: web-frontend
type: react-app
source: github.com/company/customer-portal-frontend
- name: api-backend
type: spring-boot
source: github.com/company/customer-portal-api
dependencies:
- postgres-db
- redis-cache
- name: auth-service
type: shared-service
external: true
- name: postgres-db
type: database
engine: postgresql
version: 13
- name: redis-cache
type: cache
engine: redis
deployment:
environments:
- name: development
auto_deploy: true
- name: staging
requires_approval: true
- name: production
requires_approval: true
strategy: canary
replicas: 3-10
Lessons Learned: What Makes Scaling Effective
Looking back at the evolution of web scaling, several key principles emerge that remain relevant regardless of the specific technologies used:
- Statelessness enables horizontal scaling - Moving state out of application servers remains the foundation of horizontal scalability
- Shared-nothing architectures reduce bottlenecks - Systems that don't share resources can scale more effectively
- Data locality matters - Keeping data close to computation improves performance, whether through caching or edge distribution
- Resilience requires redundancy - High availability depends on eliminating single points of failure
- Automation is essential - Manual scaling doesn't work at scale; systems must self-manage based on clear policies
- Observability drives effective scaling - You can't scale what you can't measure
- Simplicity enables reliability - Complexity is the enemy of reliability; simpler architectures are often more robust
Conclusion: The Future of Web Scaling
The history of web scaling reveals a pattern of oscillation between integration and specialization. We've moved from monolithic application servers to highly specialized components and may now be swinging back toward integrated platforms that hide complexity.
The next generation of scaling solutions may combine the best of both worlds: the simplicity and integration of earlier application servers with the flexibility, resilience, and global distribution of cloud-native architectures. The most successful platforms will likely be those that hide complexity from developers while leveraging the power of modern distributed systems.
As we look to the future, we might consider what we lost in the transition from integrated application servers to specialized components - and how we might recapture the simplicity of earlier approaches without sacrificing the flexibility and power of modern cloud architecture.